PHP is a widely-used programming language that powers numerous websites and web applications on the internet. While it is a powerful tool for developers, it can also be vulnerable to security issues if not handled properly. In this blog post, we will discuss 10 essential tips for writing secure PHP code.
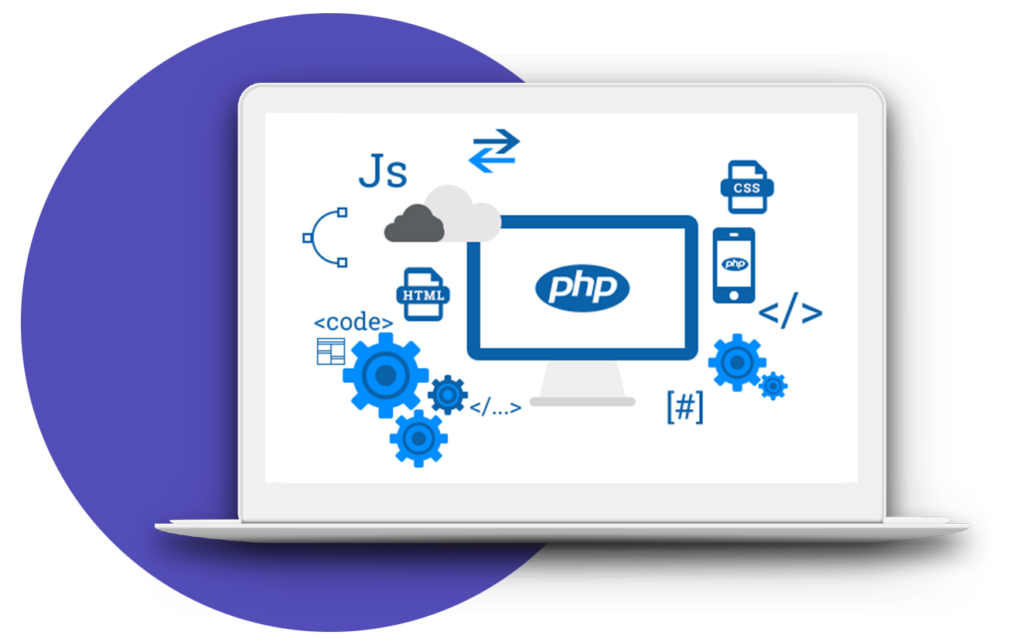
Sanitize User Input
One of the most common ways hackers exploit vulnerabilities in PHP applications is by submitting malicious code through forms or URLs. This can be prevented by sanitizing all user input and validating it before processing it. This includes checking the data type, length, and format of the input.
Use Prepared Statements for Database Queries
SQL injection attacks are another common way that hackers can exploit vulnerabilities in PHP applications. This can be prevented by using prepared statements for all database queries. Prepared statements are precompiled SQL statements that are sent to the database server with parameters. This ensures that user input is not directly included in the SQL query and thus cannot be used to execute malicious SQL code.
Use Encryption for Sensitive Data
Sensitive data such as passwords, credit card numbers, and personal information should always be encrypted. This can be done using standard encryption algorithms such as AES or RSA. PHP provides built-in functions for encrypting and decrypting data, such as openssl_encrypt() and openssl_decrypt().
Implement Access Control
Access control is the process of determining who can access certain parts of an application or database. This can be done using role-based access control (RBAC) or attribute-based access control (ABAC). RBAC assigns roles to users based on their job responsibilities, while ABAC uses attributes such as user location or job title to determine access privileges. Access control is an important part of preventing unauthorized access to sensitive data and functions.
Use a Content Security Policy (CSP)
A Content Security Policy (CSP) is an HTTP header that tells web browsers which resources are allowed to be loaded on a webpage. This can prevent cross-site scripting (XSS) attacks by limiting the sources of JavaScript, CSS, and other resources. A CSP can also prevent clickjacking attacks by limiting the frame sources that can display the page.
Use Secure Session Management
Sessions are a way for web applications to maintain state across multiple requests. However, if not managed properly, sessions can be vulnerable to attacks such as session hijacking or session fixation. To prevent these attacks, sessions should be managed securely by using unique session IDs, expiring sessions after a certain amount of time, and destroying sessions when the user logs out.
Validate File Uploads
File upload functionality is a common feature in web applications, but it can also be a security vulnerability. Hackers can use file uploads to upload malicious files such as malware or viruses. To prevent this, file uploads should be validated by checking the file type, size, and format. Files should also be stored outside of the webfoot to prevent direct access.
Disable Error Reporting
Error reporting can be useful for debugging PHP applications, but it can also reveal sensitive information such as database credentials or file paths. To prevent this, error reporting should be disabled on production servers. Instead, errors should be logged to a file or sent to a logging service.
Use a Firewall
A firewall is a network security tool that monitors and filters incoming and outgoing traffic based on predefined rules. A firewall can prevent attacks such as SQL injection, cross-site scripting, and cross-site request forgery (CSRF) by blocking malicious traffic. There are several free and paid firewall solutions available for PHP applications, such as MoD Security and Cloudflare.
Keep PHP and Libraries Up to Date
Finally, it is important to keep PHP and any third-party libraries up to date with the latest security patches. PHP vulnerabilities are regularly discovered and patched,
Mastering PHP: Best Practices and Tools for Debugging
PHP is a popular programming language that is widely used for web development. As with any programming language, there are certain best practices that developers should follow in order to write high-quality code that is easy to maintain and debug. In this blog post, we will discuss some of the best practices for mastering PHP, as well as some tools for debugging.
Use a Framework
One of the best ways to master PHP is to use a framework such as Laravel, Symfony, or CodeIgniter. A framework provides a set of tools and conventions for building web applications, which can save developers a lot of time and effort. Frameworks also help to ensure that code is written in a consistent manner, which can make it easier to maintain and debug.
Follow Coding Standards
It is important to follow coding standards when writing PHP code. This helps to ensure that code is readable and maintainable by other developers. There are several coding standards for PHP, such as the PSR-1 and PSR-2 standards, which are developed by the PHP-FIG (Framework Interop Group). Developers should also follow the coding standards set by the framework they are using.
Use Object-Oriented Programming (OOP)
Object-oriented programming (OOP) is a programming paradigm that is widely used in PHP. OOP allows developers to write code that is modular, reusable, and easier to maintain. OOP also makes it easier to write testable code, which can help to ensure that applications are bug-free.
Use Namespaces
Namespaces are a way of organizing PHP code into logical groups. Namespaces can help to prevent naming collisions between different pieces of code, which can make it easier to maintain and debug applications. Namespaces also make it easier to write reusable code that can be shared between different applications.
Use Composer
Composer is a package manager for PHP that makes it easy to manage dependencies between different pieces of code. Composer allows developers to install and update packages from the command line, which can save a lot of time and effort. Composer also makes it easy to share code between different applications.
Use a Debugger
A debugger is a tool that allows developers to step through code line-by-line, set breakpoints, and inspect variables. A debugger can be an invaluable tool for debugging PHP applications. There are several debuggers available for PHP, such as Xdebug and Zend Debugger.
Use Logging
Logging is a way of recording events that occur in an application. Logging can be a useful tool for debugging PHP applications, as it allows developers to see what is happening in an application in real-time. There are several logging libraries available for PHP, such as Monolog and Log4PHP.
Use PHP Unit
PHP Unit is a unit testing framework for PHP that allows developers to write automated tests for their code. Automated tests can help to ensure that applications are bug-free and can save a lot of time and effort in the long run. PHP Unit allows developers to write tests for their code in a consistent and repeatable manner.
Use a Profiler
A profiler is a tool that allows developers to see how much time their code is spending on different tasks. A profiler can be a useful tool for identifying performance bottlenecks in PHP applications. There are several profilers available for PHP, such as XHProf and Blackfire.io.
Use Version Control
Version control is a way of managing changes to code over time. Version control can help to ensure that code is written in a consistent manner and can make it easier to debug applications. There are several version control systems available for PHP, such as Git and Subversion.
In conclusion, mastering PHP requires following best practices, such as using a framework, following coding standards, and using OOP.
The Future of PHP: An Overview of PHP 8 Features and Improvements
PHP is one of the most popular programming languages for web development. It has been around since 1994 and has gone through several iterations, with the latest major release being PHP 8. In this blog post, we will provide an overview of the new features and improvements in PHP 8 and discuss the future of PHP.
Just-In-Time (JIT) Compiler
One of the most significant new features in PHP 8 is the addition of a JIT compiler. This feature can improve performance by up to 30%, depending on the application. The JIT compiler dynamically generates native machine code at runtime, which can significantly speed up the execution of code.
Union Types
Union types are a new type declaration that allows developers to specify that a variable can be one of several types. This can make code more concise and easier to read. Union types can also help to prevent errors, as they provide a way to explicitly declare what types of data a function expects.
Named Arguments
Named arguments are a new feature that allows developers to pass arguments to a function by name, rather than by position. This can make code more readable and can help to prevent errors caused by passing arguments in the wrong order.
Match Expression
Match expressions are a new control structure that provides a more concise and readable way to write complex conditional statements. They are similar to switch statements, but with a more flexible syntax that allows for more complex matching.
Attributes
Attributes are a new way to add metadata to classes, functions, and other parts of the code. Attributes can be used to provide information about the code to IDEs and other tools, and can also be used to trigger specific behaviors in the code.
Building Dynamic Web Applications with PHP and MySQL: A Beginner’s Guide
PHP is a popular server-side scripting language used for building dynamic web applications, and MySQL is a widely used open-source database management system. Together, they provide a powerful platform for building modern, data-driven web applications. In this beginner’s guide, we will explore the basics of building dynamic web applications with PHP and MySQL.
What is a Dynamic Web Application?
A dynamic web application is a web application that generates content dynamically in response to user input or other events. A dynamic web application typically uses a combination of server-side scripting, client-side scripting, and database access to generate content on-the-fly. Some examples of dynamic web applications include social media sites, online marketplaces, and content management systems.
Setting up the Environment
Before we begin building our dynamic web application, we need to set up the environment. We will need a web server, a database management system, and a text editor or an integrated development environment (IDE) to write our code.
There are several options available for setting up a PHP and MySQL environment, including XAMPP, WAMP, and MAMP. These are all pre-configured packages that include Apache, PHP, MySQL, and other tools. Alternatively, we can install each component separately.
Creating a Database
The first step in building a dynamic web application with PHP and MySQL is to create a database. We can use the MySQL command-line client or a graphical user interface (GUI) such as phpMyAdmin to create our database.
We will need to create a database, one or more tables, and define the fields for each table. For example, if we were building an e-commerce site, we might create a database called “store” and a table called “products” with fields for “product ID”, “product name”, “product description”, “price”, and “image”.
Connecting to the Database
Once we have our database set up, we need to connect to it from our PHP code. We can use the mysqli or PDO extensions to connect to the database and execute SQL queries.
We will need to provide the database hostname, username, password, and database name to establish a connection. Once the connection is established, we can execute SQL queries to insert, update, delete, or retrieve data from the database.
Displaying Data on the Webpage
Now that we can connect to the database, we can display data on the webpage. We can use PHP to query the database and generate HTML to display the data.
For example, if we wanted to display a list of products on our e-commerce site, we might use a loop to retrieve the product data from the database and generate HTML to display the product name, description, price, and image.
Adding User Authentication
Most dynamic web applications require some form of user authentication to protect sensitive data and prevent unauthorized access. We can use PHP and MySQL to implement user authentication.
We will need to create a user table in the database and store the user’s username and password in the table. We can then use PHP to check the user’s credentials and grant access to protected areas of the site.
Adding Interactivity with JavaScript
To make our dynamic web application more interactive, we can use JavaScript to add client-side interactivity. For example, we might use JavaScript to validate user input, display pop-up dialogs, or update the page without reloading.
JavaScript can also be used to send requests to the server using AJAX (Asynchronous JavaScript and XML) to retrieve data from the server without reloading the entire page.